Structure of a C++ program
Topic asked in February 2022 (CBCS) , December 2022 (CBCS) , July 2022 (CBCS) and March 2021 (CBCS) question paper.
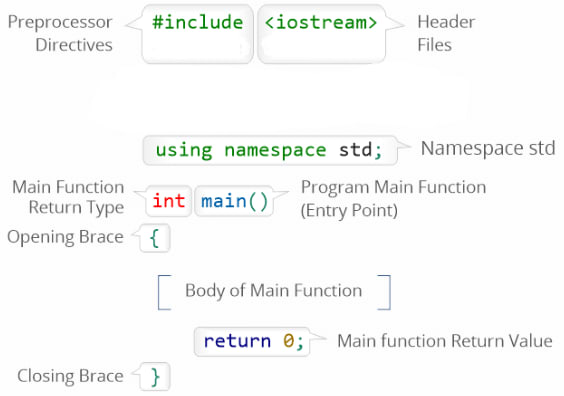
A C++ program consists of declarations and definitions, organized into files that are combined through linking after separate compilation. It is divided into three main sections:
- Standard Libraries Section: This section contains the header files for the standard libraries that your program will use.
- Main Function Section: This section contains the main function, which is the entry point for your program.
- Function Body Section: This section can contain definitions for functions used in the program, including main.
Standard Libraries Section
- The
#include
directive is a preprocessor directive used to include header files in C++ programs. Header files are important in C++ because they store predefined functions and data types, which make programming easier. They can reduce the complexity and number of lines of code, and allow for reusing functions in different .cpp files. <iostream>
specifically imports the input-output stream library, which provides functionality for input and output operations in C++.namespace std
specifies that names likecout
andendl
are defined within thestd
namespace and can be used in the program.
Main Function Section
- The main() function is the entry point of a C++ program, where execution begins.
- The curly brace
{
marks the beginning of the main function body. - The curly brace
}
marks the end of the main function body. - It returns an integer value (int), typically 0 to indicate successful execution.
Function Body Section
- cout is used to display output to the console, and
<<
is the stream insertion operator. - "Hello, Everyone" is the text to be displayed, followed by
endl
to insert a newline and flush the output buffer. - return 0; terminates the main() function, returning 0 to the operating system to indicate successful execution.
Here is full code:
Output:
This code demonstrates a simple C++ program that prints "Hello, Everyone" to the console.
How's article quality?