Expression In C++
An expression is a combination of operators, constants and variables. An expression may consist of one or more operands, and zero or more operators to produce a value.
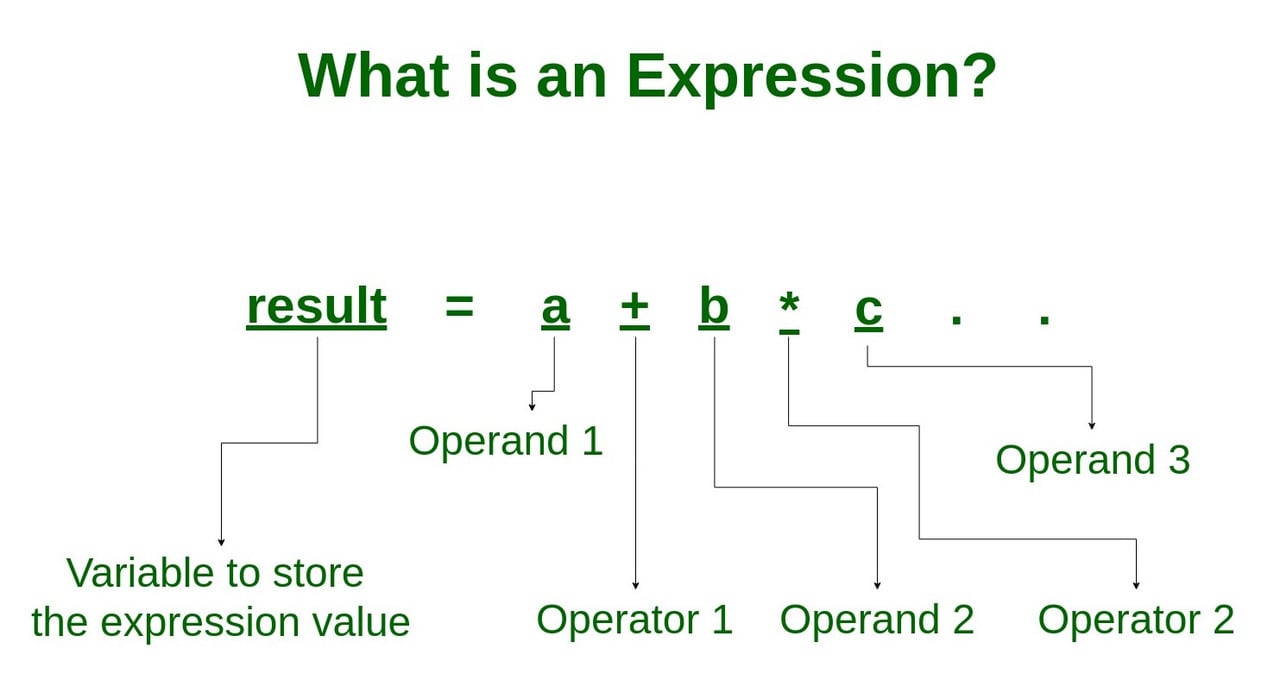
Types Of Expressions
-
Constant expressions
Constant Expressions consists of only constant values. A constant value is one that doesn't change. It is an expression whose value is determined at the compile-time. It can be composed of integer, character, floating-point, and enumeration constants.
Example:-
Output:-
-
Integral expressions
Integral Expressions are those which produce integer results after implementing all the automatic and explicit type conversions.
Example:-
Output:-
-
Float expressions
Float Expressions are which produce floating point results after implementing all the automatic and explicit type conversions.
Example:-
Output:-
-
Pointer expressions
This particular pointer expression in C++ produces an address value as an output of the program.
Example:-
Output:-
-
Relational expressions
A relational expression is an expression that produces a value of type bool, which can be either true or false. It is also known as a boolean expression. When arithmetic expressions are used on both sides of the relational operator, arithmetic expressions are evaluated first, and then their results are compared.
Example:-
Output:-
-
Logical expressions
Logical Expressions combine two or more relational expressions and produces bool type results.
Example:-
Output:-
-
Bitwise expressions
Bitwise Expressions are used to manipulate data at bit level. They are basically used for testing or shifting bits.
Example:-
Output:-
-
Special assignment expressions
Special assignments in C++ can be classified depending on the value of the variable that is assigned
-
Chained assignment expressions - In chained assignment expression assigns the same values more than once by using only one statement
Example:-
Output:-
-
Embedded assignment expressions - In this embedded assignment expression one expression is enclosed within another assignment expressions
Example:-
Output:-
-
Compound Assignment - A compound assignment expression is an expression which is a combination of an assignment operator and bioy operator.
Example:-
Output:-
-
Last updated on -