Conditional Statements
Conditional statements in C++ are programming constructs that allow the execution of different blocks of code based on the evaluation of conditions. They help control the flow of execution in a program by determining whether certain blocks of code should be executed or skipped.
There are primarily two types of conditional statements in C++:
- if-else Statement
- Switch Statement
If-Else Statements
The if-else
statement in C++ allows you to execute a block of code based on the evaluation of a condition.
If the condition is true, the code inside the if
block is executed; otherwise, the code inside the else
block is executed.
Here's the general syntax:
If the condition specified inside the if
parentheses evaluates to true, the code block inside the if
statement is executed.
If the condition evaluates to false, the code block inside the else
statement (if present) is executed.
Example:-
Output:-
Else-If
The else if
statement is an extension of the if-else
statement in C++.
It allows you to specify additional conditions to be evaluated if the preceding
if condition (or any previous else if
condition) evaluates to false.
This allows for more complex decision-making within your code.
Here's the general syntax of the else if
statement:
You can have multiple else if
blocks after an initial if
statement. Each else if
block is only evaluated if all preceding conditions
(including the if
condition and any previous else if
conditions) are false.
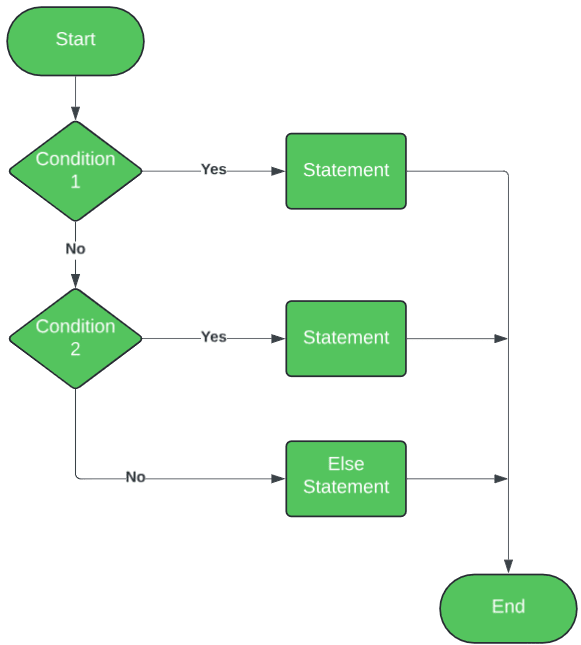
Code Example:
Output:
Nested If-Else
Topic asked in July 2022 (CBCS) and December 2022 (CBCS) question paper.
Nested if-else
statements are used when you need to check multiple conditions sequentially, where one condition depends on the outcome of another condition.
In C++, you can nest if
statements within other if
or else
blocks to create more complex conditions.
This nesting can occur within both if
and else
portions of the main if
statement.
Here's the general structure of nested if
statements:
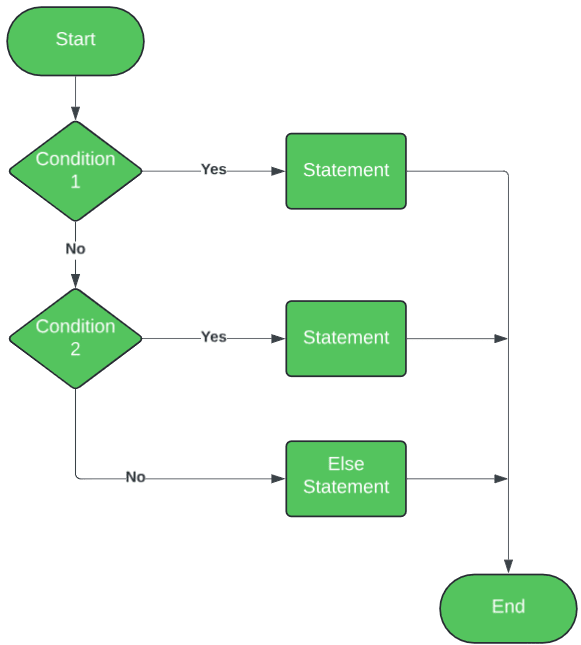
Code Example:- Check the greatest of three numbers
Output:-
Explanation:-
- Three integers x, y, and z are declared with values 9, 7, and 5 respectively.
- The outermost if-else statement checks if x is less than y.
- Since x (9) is greater than y (7), the else block is executed.
- Inside the else block, another if-else statement checks if z is less than x.
- Since z (5) is less than x (9), the if block is executed, and it prints "9 is the greatest".
Switch Statements
Topic asked in February 2022 (CBCS) and July 2022 (CBCS) question paper.
In C++, a switch
statement is a control flow statement used to select one of many code blocks to be executed based on the value of an expression.
It's particularly useful when you have multiple cases to handle and want a more elegant alternative to using nested if
statements.
Here's the basic syntax of a switch
statement in C++:
Here's a breakdown of each component:
-
expression: This is typically a variable or an expression whose value will be compared against the values specified in the case labels.
-
case value1: This is a label that specifies one of the possible values of the expression. If the value of the expression matches value1, the code block following this label will be executed.
-
break: This statement is used to exit the switch block. If a break statement is not included, execution will "fall through" to the next case, executing its code block as well.
-
default: This is an optional label that provides a default case in case none of the specified cases match the value of the expression. It is executed when none of the case labels match the expression.
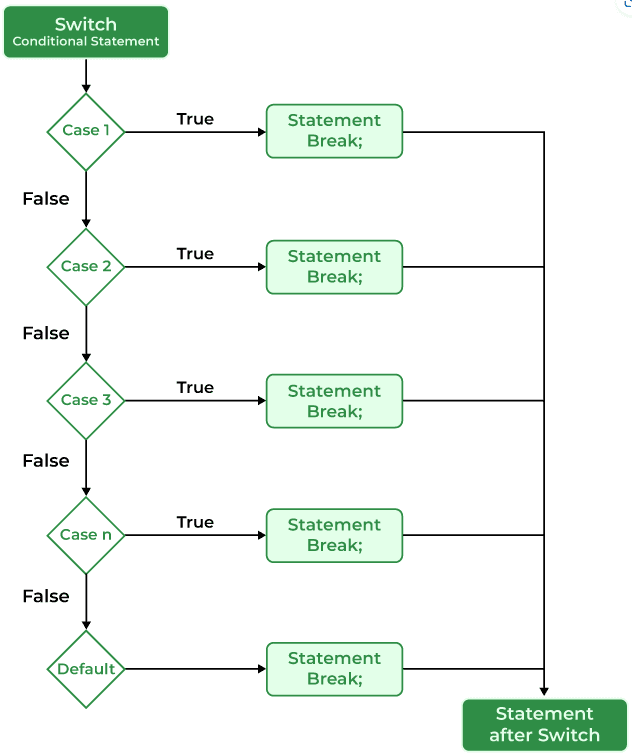
Here's a simple example in C++ to illustrate how a switch statement works:
Output:-
Nested Switch Case
Nested switch cases in C++ refer to the situation where a switch
statement is nested within another switch statement.
This is useful when you need to make a decision based on multiple criteria, with each criterion having multiple options.
Here's an example of nested switch
cases:
Output:-
Let's break down how this nested switch
case works:
- The program prompts the user to enter two options.
- The first
switch
statement checks the value ofoption1
. - Inside each
case
of the outerswitch
statement, there's anotherswitch
statement nested, which checks the value ofoption2
. - Depending on the combination of
option1
andoption2
, the program prints a corresponding message.
Last updated on -