Control Flow Statements in Python
Control flow statements are essential in programming as they determine the order in which instructions are executed. In Python, control flow is managed through sequential, decision, and loop control flow statements. Understanding these is key to writing effective and efficient code.
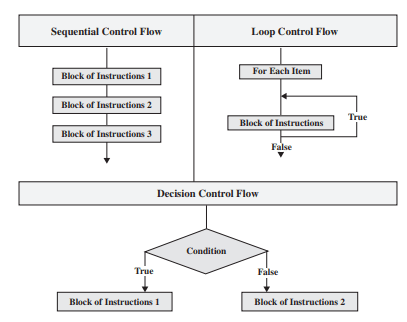
Sequential Control Flow Statements - Sequential control flow is the default mode of execution in Python, where statements are executed one after another in the order they appear.
Decision Control Flow Statements - Decision control flow statements allow your program to choose different paths of execution based on conditions. The primary decision control statements in Python are if, elif, and else.
Loop Control Flow Statements - Loop control flow statements are used to execute a block of code multiple times. Python supports two main types of loops: for and while.
Decision Control Flow Statements in Python
Decision control flow statements in Python allow you to make choices in your code,
executing different blocks of code based on conditions.
The primary decision control flow statements are if
, if...else
, if...elif...else
, and nested if
statements.
Let's delve into each type of decision control flow statement in detail.
if
Statement
The if
statement is the most basic form of decision-making in Python.
It evaluates a condition (an expression that returns a boolean value, either True
or False
).
If the condition evaluates to True
, the block of code within the if
statement is executed.
If the condition is False
, the code block is skipped.
Syntax:
Example:
In this example, if temperature
is greater than 30, the message "It's a hot day!" is printed.
if...else
Statement
The if...else
statement provides an alternative path of execution. If the if
condition evaluates to False
,
the code inside the else
block is executed.
Syntax:
Example:
In this example, if age
is 18 or older, "You are an adult." is printed. Otherwise, "You are a minor." is printed.
if...elif...else
Statement
The if...elif...else
statement allows for multiple conditions to be evaluated sequentially.
Each elif
(short for "else if") block is checked only if all previous conditions were False
.
The else
block executes if none of the preceding conditions are True
.
Syntax:
Example:
In this example, the program checks the score
and prints Grade: B.
Nested if
Statements
Nested if
statements are if
statements placed inside another if
statement. This allows for more complex conditions to be evaluated.
Syntax:
Example:
In this example, the program first checks if age
is 18 or older. If true, it then checks if has_ticket
is True
. Depending on these conditions, appropriate messages are printed. Here it will print "You can enter the movie theater".
Loop Control Flow Statements in Python
Loop control flow statements in Python allow you to execute a block of code repeatedly. This repetition can be controlled in several ways using while
loops, for
loops, and by utilizing continue
and break
statements to manage the flow within loops.
The while
Loop
The while
loop repeatedly executes a block of code as long as a specified condition evaluates to True
. It is commonly used when the number of iterations is not known before the loop starts.
Syntax:
Explanation:
condition
: An expression that is evaluated before each iteration. If it evaluates toTrue
, the loop body is executed. If it evaluates toFalse
, the loop terminates.- The loop body should include code that modifies the condition or otherwise influences when the loop should end to avoid infinite loops.
Example:
Output:
In this example, the loop will continue to execute as long as count
is less than 5. With each iteration, count
is incremented by 1, eventually making the condition False
and terminating the loop.
The for
Loop
The for
loop is used to iterate over a sequence (such as a list, tuple, dictionary, string, or range) or other iterable objects. It is typically used when the number of iterations is known or can be easily determined.
Syntax:
Explanation:
variable
: A name that takes on the value of each item in the sequence on each iteration.sequence
: An iterable object that is being iterated over. This could be a list, tuple, string, or any object that supports iteration.
Example:
Output:
In this example, range(5)
generates numbers from 0 to 4. The for
loop iterates over these numbers, and each number is printed.
The continue
Statement
The continue
statement is used to skip the current iteration of a loop and proceed to the next iteration. When continue
is executed, the remaining code in the loop body is skipped for the current iteration.
Syntax:
Example:
Output:
In this example, the continue
statement skips the print statement for even numbers, resulting in only odd numbers being printed.
The break
Statement
The break
statement is used to exit a loop prematurely. When break
is executed, the loop is terminated, and the program control moves to the first statement after the loop.
Syntax:
Example:
Output:
In this example, the loop will terminate when i
equals 5. Therefore, only numbers from 0 to 4 are printed before the loop exits.
Combining continue
and break
Statements
You can use continue
and break
statements together within the same loop to control the flow more precisely.
Example:
Output:
In this example:
continue
skips even numbers.break
exits the loop wheni
equals 7.- Numbers 1, 3, and 5 are printed before the loop terminates.